数组
练习:Three Sum(求三数之和)
思路:
- 先将数组排序。
- 将Three Sum转换为Two Sum来做。
将num[i]的相反数即-num[i]作为target,然后从i+1到len(num)-1的数组元素中寻找两个数使它们的和为-num[i]。下标i的范围是从0到len(num)-3。 - 过程中注意去重。(否则会报错:Time Limit Exceeded)
- 时间复杂度为O(N^2)。
1 | class Solution: |
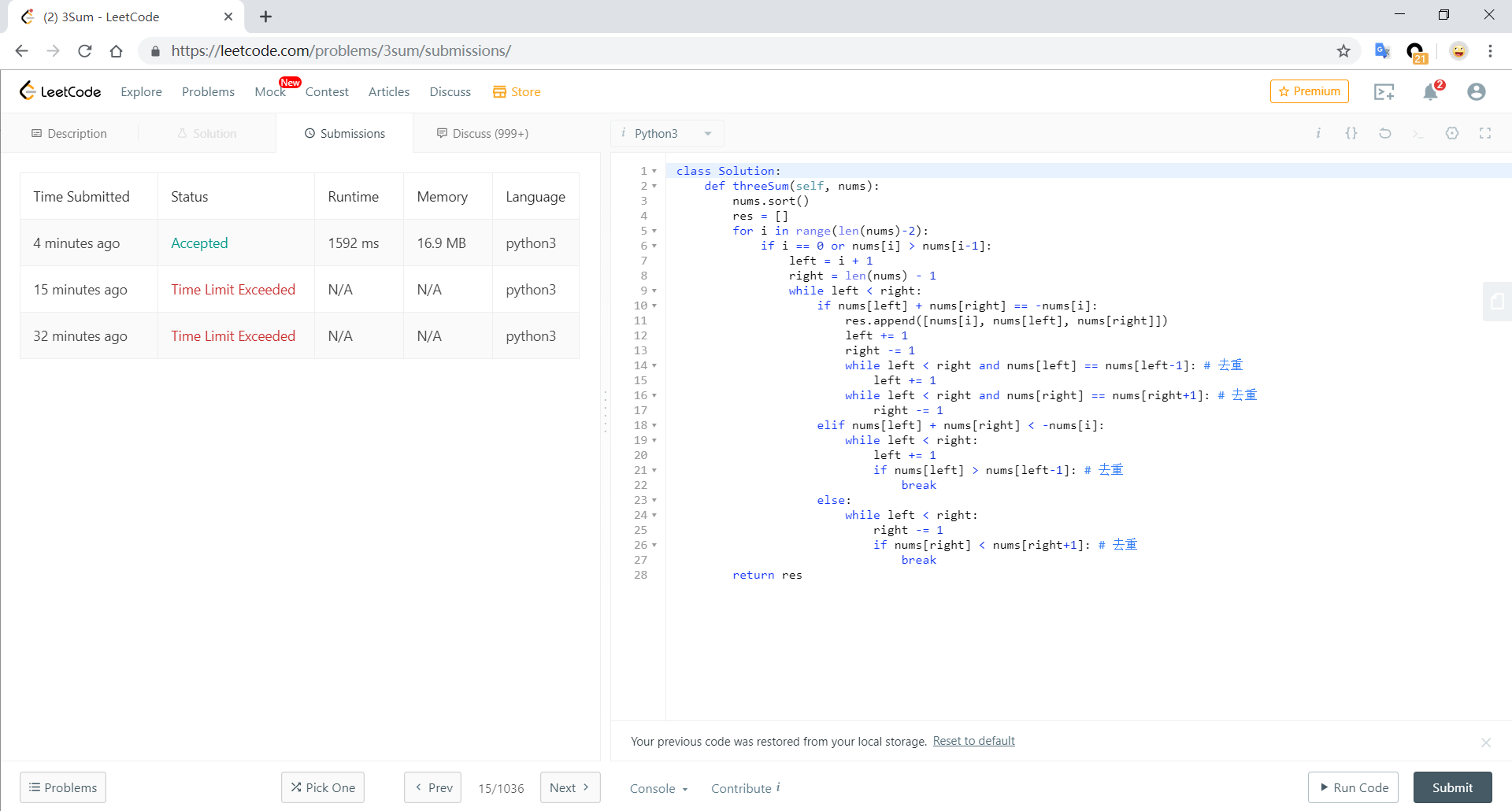
Majority Element(求众数)
思路:
按列表去重后的元素依次计数、比较。
1 | class Solution: |
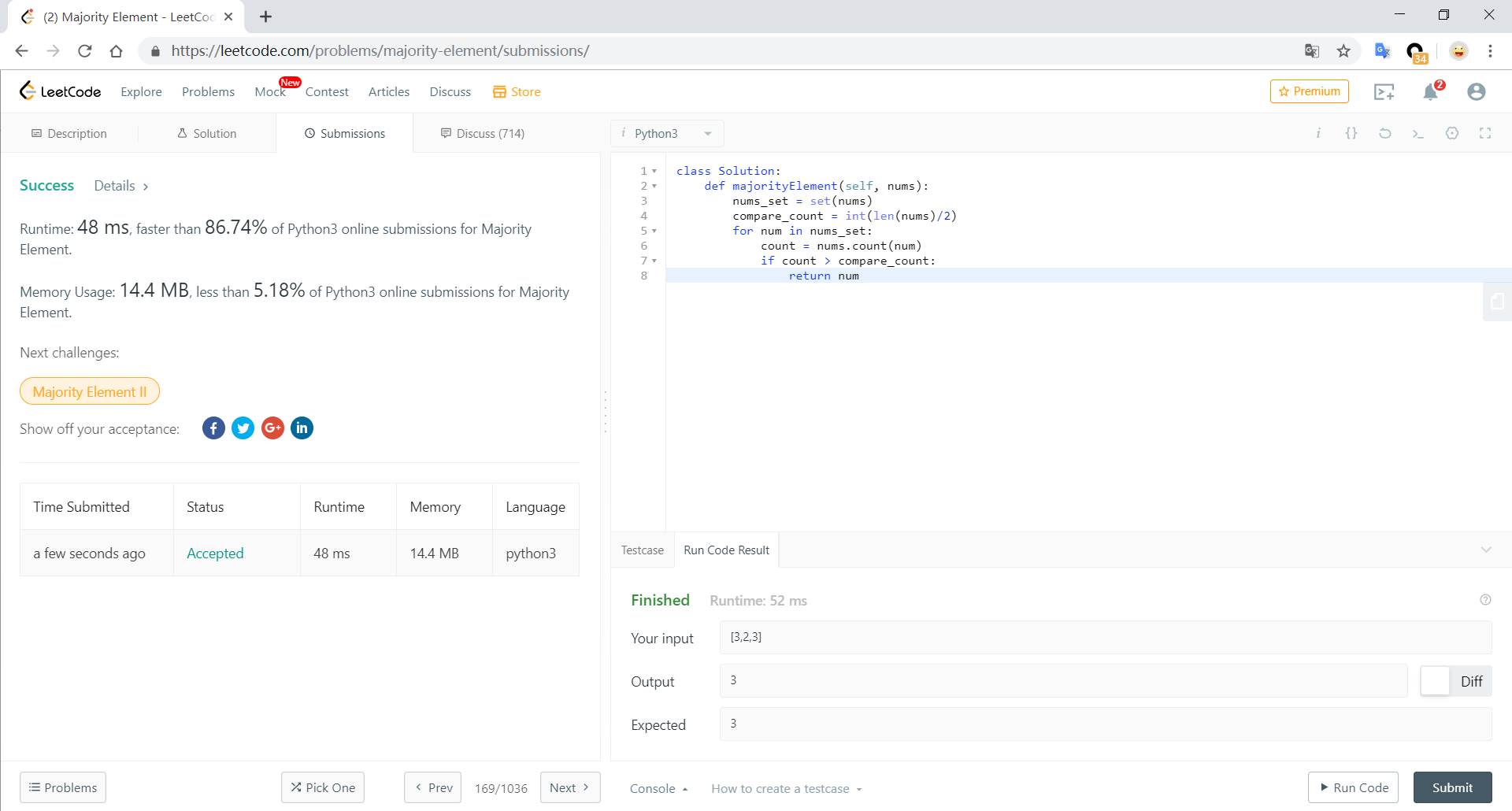
Missing Positive(求缺失的第一个正数)
要求:时间复杂度在O(n)内,使用恒定的额外空间。
思路:
使用字典hash求解。
1 | class Solution: |
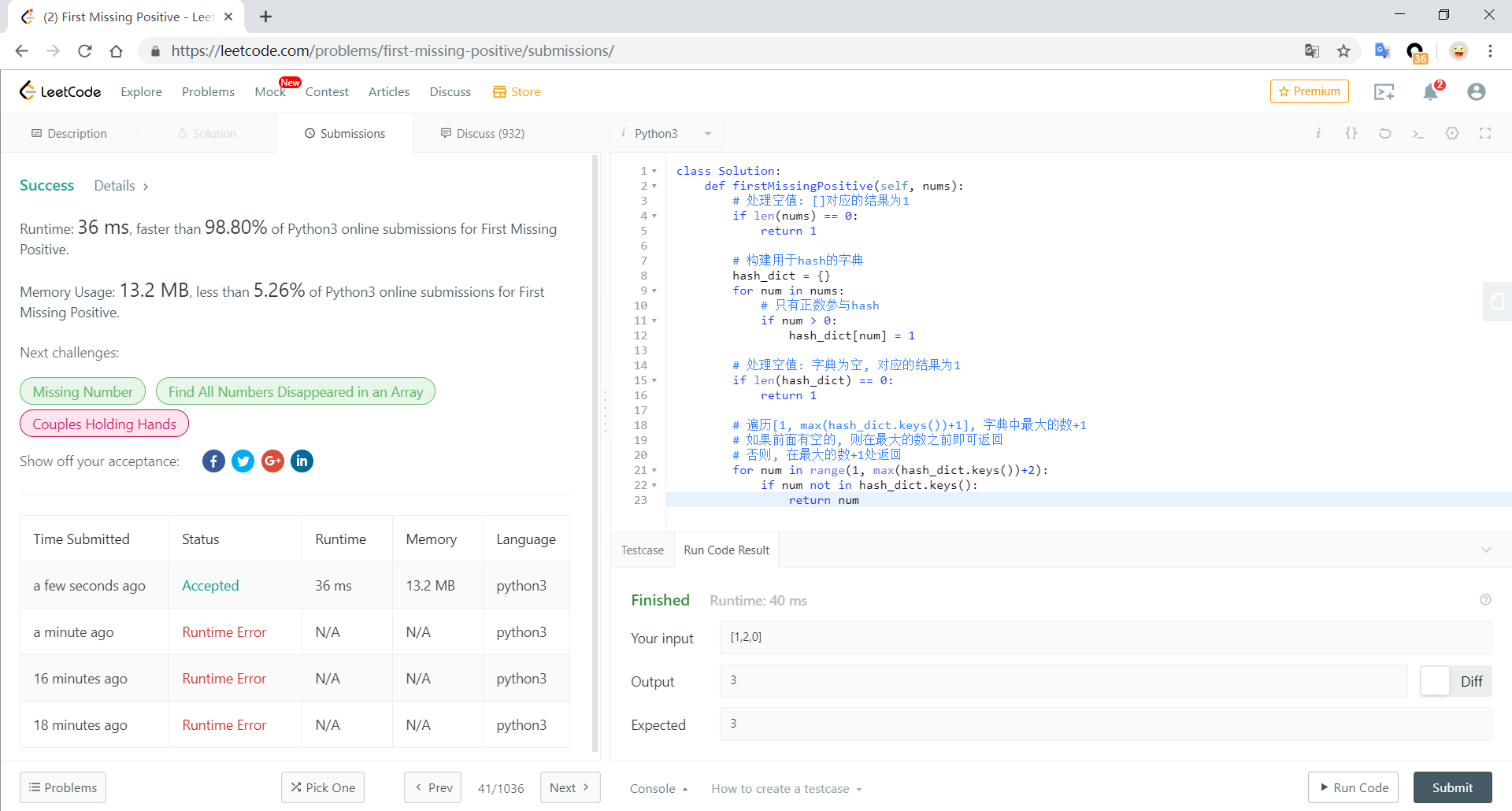
链表
练习:Linked List Cycle I(环形链表)
思路:快慢指针1
2
3
4
5
6
7
8
9class Solution(object):
def hasCycle(self, head):
slow = fast = head
while fast and fast.next:
fast = fast.next.next
slow = slow.next
if slow == fast:
return True
return False
Merge k Sorted Lists(合并 k 个排序链表)
思路:小根堆保存值和索引1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19class Solution:
def mergeKLists(self, lists):
head = ListNode(-1)
move = head
heap = []
heapq.heapify(heap)
[heapq.heappush(heap, (l.val, i)) for i, l in enumerate(lists) if l]
while heap:
curVal, curIndex = heapq.heappop(heap)
curHead = lists[curIndex]
curNext = curHead.next
move.next = curHead
curHead.next = None
move = curHead
curHead = curNext
if curHead:
lists[curIndex] = curHead
heapq.heappush(heap, (curHead.val, curIndex))
return head.next
相关链接
重磅 | 完备的 AI 学习路线,最详细的资源整理!
Datawhale第七期组队学习计划
编程计划说明
编程计划的第1个任务
第一次任务 打卡表格